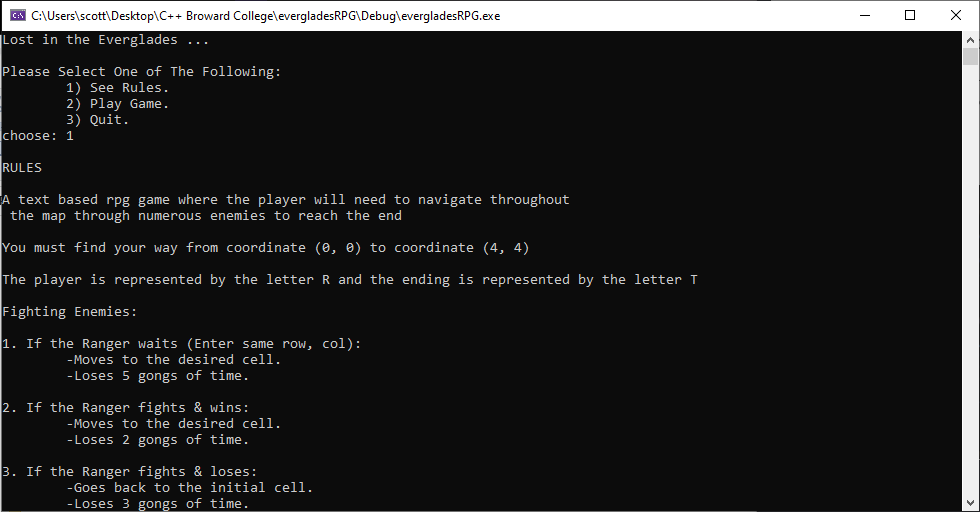
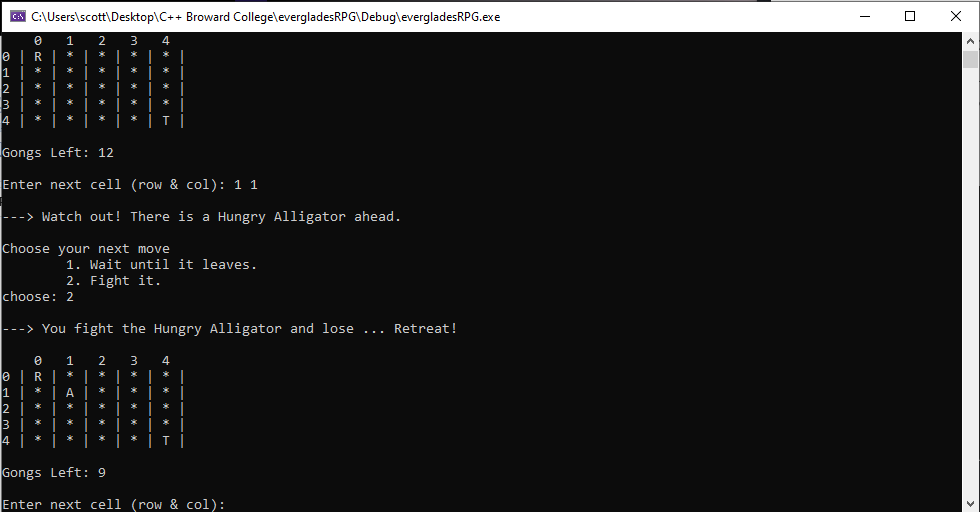
×
/*
Scott Hernandez
evergladesRPG.cpp
Description: This application is a text based rpg game. You have to fight your way through enemies to get
to the end while staying alive.
Input: Player must go through a variety of prompts entering whether to read the rules, begin the game,
quit, coordinates to their next movement, whether to fight or run
Process: 1. Prompt shows up asking player whether to
- show rules (Shows rules about how to play the game)
- play (Starts the game)
- quit (Quits the game)
2. When player selects to Play they have a certain amount of life and given
the option of where they would like to move on the map
- The map is generated through a for loop(map that is displayed) as well as the enemies
map
- The game loop beings after generation of maps
3. When moving throughout the map, the player will find enemies along the tiles and can
choose whether to fight or wait for the enemy to move.
- Choosing to fight will either make you advance losing minimal gongs(life) or lose and
get reset on the map
- Choosing to wait will greatly effect your gongs(life)
4. Players that reach the end will be prompted with a message and prompted again with the initial
prompts of showing rules, playing again, or quitting
- Game loop is broken out of and the program is restarted
Output: text based coordinate system map style game will be displayed.
*/
// Header Files
#include <iostream>
#include <string>
#include <iomanip>
#include <ctime>
#include <cstdlib>
using namespace std;
// Prototype Functions
void printStartMenu();
void checkStartMenuOption(int, bool&);
void printRules();
int checkSpace(int, int, int, string const[]);
int fightingPrompt(int, int);
void getMovement(int&, int&, int&, int&);
void gameOverMessage(int&);
int fightingPromptActions(int, int&, string, int&, int&, int&, int, int, int&);
void checkGameOver(int, int, int, bool&);
void setTopCoordinates();
// Main Function
int main() {
//||||||||||||||||||||||||||||||||||||||||||||||||||||||||\\
// \\
// \\
// Variables \\
// \\
//||||||||||||||||||||||||||||||||||||||||||||||||||||||||\\
int const FREE = 0, // Constants to tell us what is on each tile
PLAYER = 1,
HUNGRY_ALLIGATOR = 2, SWARM_OF_GIANT_MOSQUITOS = 3, VENOMOUS_SPIDER = 4, PYTHON = 5, END = 6,
ENEMY = 1;
int gongs = 12; // Gongs(life) left
int chosenCol, chosenRow; // user inputted location values
char const TYPES[] = { '*', 'R', 'A', 'M', 'S', 'P', 'T' }; // Map value single characters
string const ENEMIES[] = { "Hungry Alligator", "Swarm of Giant Mosquitos", "Venomous Spider", "Python" }; // Enemy names in string value
int map[5][5]; // Game map
int enemiesMap[5][5]; // Hidden enemy map
int player_x = 0, player_y = 0; // player x and y
//||||||||||||||||||||||||||||||||||||||||||||||||||||||||\\
// \\
// \\
// Beginning of Program \\
// \\
//||||||||||||||||||||||||||||||||||||||||||||||||||||||||\\
cout << "Lost in the Everglades ..." << endl << endl; // Start of program message
// Start Menu
printStartMenu();
//||||||||||||||||||||||||||||||||||||||||||||||||||||||||\\
// \\
// \\
// Creation of Map \\ Creator: Scott Hernandez
// \\
//||||||||||||||||||||||||||||||||||||||||||||||||||||||||\\
for (int i = 0; i < 5; i++) // Loop through map and set each tile to value of FREE
{
for (int j = 0; j < 5; j++)
{
map[i][j] = FREE;
}
}
map[player_x][player_y] = PLAYER; // Set the starting position of the player
map[4][4] = END; // Set the position of the ending tile
//||||||||||||||||||||||||||||||||||||||||||||||||||||||||\\
// \\
// \\
// Generation of Enemies Map \\ Creator: Scott Hernandez
// \\
//||||||||||||||||||||||||||||||||||||||||||||||||||||||||\\
for (int i = 0; i < 5; i++) // Set up enemy map to values of map tiles
{
for (int j = 0; j < 5; j++)
{
enemiesMap[i][j] = map[i][j];
}
}
int amount = 0;
srand(unsigned(time(0)));
for (int i = 0; i < 5; i++) // Loop through each tile and set 10 enemies on the map randomly
{
for (int j = 0; j < 5; j++)
{
if (enemiesMap[i][j] == 0 && amount < 10)
{
int setEnemy = rand() % 2;
int r = (rand() % 4) + 2;
if (setEnemy == 0)
{
enemiesMap[i][j] = r;
amount++;
}
}
}
}
//||||||||||||||||||||||||||||||||||||||||||||||||||||||||\\
// \\
// \\
// Start of Game Loop \\
// \\
//||||||||||||||||||||||||||||||||||||||||||||||||||||||||\\
bool running = true; // While game is running
while (running) { // Loop until game ends
/*
*
* SHOW MAP
*
* */
setTopCoordinates();
for (int i = 0; i < 5; i++) { // Loop through map and display map in console
cout << i << " ";
cout << "| ";
for (int j = 0; j < 5; j++) {
string value = " ";
if (map[i][j] != -1)
value = TYPES[map[i][j]];
cout << value << " | ";
}
cout << endl;
}
/*
*
* DISPLAY GONGS
*
* */
cout << "\nGongs Left: " << gongs << endl << endl; // Gongs left
/*
*
* PROMPT FOR USER NEXT CELL MOVEMENT
*
* */
getMovement(player_x, player_y, chosenRow, chosenCol); // Get player movement user selected values
/*
*
* CHECK SPACE
*
* */
int spaceCheck = 0;
if (chosenCol == player_y && chosenRow == player_x) // Check space that user selects
cout << endl << "You are already standing there!" << endl << endl;
else
spaceCheck = checkSpace(enemiesMap[chosenRow][chosenCol], chosenRow, chosenCol, ENEMIES);
/*
*
* FIGHTING PROMPT
*
* */
int move = fightingPrompt(spaceCheck, ENEMY); // Prompt fighting prompt if user finds an enemy
/*
*
* FIGHTING PROMPT ACTIONS
*
* */
// Check enemy space
int space = enemiesMap[chosenRow][chosenCol];
// Execute fighting prompt actions and then set players positioning on map as well as enemy positioning based on returned value
int f = fightingPromptActions(move, enemiesMap[player_x][player_y], ENEMIES[space - 2 || 0], map[player_x][player_y], player_x, player_y, chosenRow, chosenCol, gongs);
if (f == 0) // player has lost and is set back to spawn
{
map[chosenRow][chosenCol] = space; // Show enemy on visible map
map[player_x][player_y] = -1; // Remove user from tile
player_x = 0; // Set player x
player_y = 0; // Set player y
map[player_x][player_y] = PLAYER; // Set player tile
}
else if (f == 1) // player has won and advances
{
enemiesMap[player_x][player_y] = FREE; // Set enemy map tile to FREE
map[player_x][player_y] = PLAYER; // Set player tile
}
/*
*
* LAST CHECKS BEFORE LOOPING AGAIN
*
* */
if (spaceCheck == FREE) { // Set location of player on map
map[player_x][player_y] = -1; // Remove player from map
player_x = chosenRow; // Set player x
player_y = chosenCol; // Set player y
map[player_x][player_y] = 1; // Set player tile
}
checkGameOver(gongs, spaceCheck, END, running); // check for game over
}
//||||||||||||||||||||||||||||||||||||||||||||||||||||||||\\
// \\
// \\
// GAME OVER \\
// \\
//||||||||||||||||||||||||||||||||||||||||||||||||||||||||\\
gameOverMessage(gongs); // Game over message
main(); // Restart game
return 0;
}
//||||||||||||||||||||||||||||||||||||||||||||||||||||||||\\
// \\
// \\
// FUNCTIONS \\
// \\
//||||||||||||||||||||||||||||||||||||||||||||||||||||||||\\
/*
void printStartMenu()
Prints the initial menu for the program and validates the users
response.
Return Value: None
Creator: Scott Hernandez
*/
void printStartMenu() {
bool startMenu = true;
int startMenuOption = 0;
while (startMenu) { // Start of menu loop
cout << "Please Select One of The Following: " << endl
<< "\t1) See Rules." << endl
<< "\t2) Play Game." << endl
<< "\t3) Quit." << endl
<< "choose: ";
cin >> startMenuOption; // Check menu value
if (cin) { // If value is a number
checkStartMenuOption(startMenuOption, startMenu); // Call function to check options
}
else {
cout << endl << "Please enter a valid value!" << endl; // Ask for another value and loop again
cin.clear();
cin.ignore(100, '\n');
}
}
cout << endl;
}
/*
void checkStartMenuOption(int option, bool& startMenu)
Depending on start menu options the switch case goes through
the options and executes code.
Return Value: None
Creator: Scott Hernandez
*/
void checkStartMenuOption(int option, bool& startMenu) {
switch (option) { // Switch case statement to check through start menu options
case 1:
// Show Rules
printRules(); // Print rules
break;
case 2:
startMenu = false; // Start game
break;
case 3:
cout << endl << "Good Bye ..." << endl; // Good bye message
exit(0);
break;
default:
cout << endl << "Please enter a valid value!" << endl;
}
}
/*
void printRules()
Prints game rules to the console
Return Value: None
Creator: Scott Hernandez
*/
void printRules() {
// Print Rules
cout << endl << "RULES" << endl << endl;
cout << "A text based rpg game where the player will need to navigate throughout" << endl
<< " the map through numerous enemies to reach the end" << endl << endl;
cout << "You must find your way from coordinate (0, 0) to coordinate (4, 4)" << endl << endl;
cout << "The player is represented by the letter R and the ending is represented by the letter T" << endl;
cout << endl << "Fighting Enemies:" << endl << endl;
cout << "1. If the Ranger waits (Enter same row, col):" << endl
<< "\t-Moves to the desired cell." << endl
<< "\t-Loses 5 gongs of time." << endl;
cout << "\n2. If the Ranger fights & wins:" << endl
<< "\t-Moves to the desired cell." << endl
<< "\t-Loses 2 gongs of time." << endl;
cout << "\n3. If the Ranger fights & loses:" << endl
<< "\t-Goes back to the initial cell." << endl
<< "\t-Loses 3 gongs of time." << endl
<< "\t-The Danger remains in the cell." << endl << endl;
}
/*
int checkSpace(int space, int x, int y, string const ENEMIES[])
Checks each space the player wants to move to see if the space
has someone on it, is free, or has an enemy on it and returns the value.
Return Value: Returns space value
Creator: Scott Hernandez
*/
int checkSpace(int space, int x, int y, string const ENEMIES[]) {
switch (space) { // Switch case statement to check the space passed in
case 0: // FREE SPACE
cout << endl << "--> Cell (" << x << ", " << y << ") is Free ... You Advance!" << endl << endl;
return 0;
break;
case 2: // HUNGRY_ALLIGATOR
cout << "\n---> Watch out! There is a " + ENEMIES[0] + " ahead." << endl;
return 1;
break;
case 3: // SWARM_OF_GIANT_MOSQUITOS
cout << "\n---> Watch out! There is a "+ ENEMIES[1] +" ahead." << endl;
return 1;
break;
case 4: // VENOMOUS_SPIDER
cout << "\n---> Watch out! There is a " + ENEMIES[2] + " ahead." << endl;
return 1;
break;
case 5: // PYTHON
cout << "\n---> Watch out! There is " + ENEMIES[3] + " ahead." << endl;
return 1;
break;
case 6:
cout << endl << "---> Congratulations! You have made it to the end!" << endl;
return 6;
break;
}
return 0;
}
/*
int fightPrompt(int spaceCheck, int TYPE)
Prompts fight prompt when user steps on a space with an enemy
and returns the value the user selects
Return Value: Selected fighting prompt value
Creator: Scott Hernandez
*/
int fightingPrompt(int spaceCheck, int TYPE) {
bool enemyFound = false;
if (spaceCheck == TYPE) // if spaceCheck is equal to an enemy
enemyFound = true;
int move = 0;
while (enemyFound) { // Loop through fighting prompt
cout << endl << "Choose your next move" << endl
<< "\t1. Wait until it leaves." << endl
<< "\t2. Fight it." << endl
<< "choose: ";
cin >> move; // Check for move
while (!cin || (move < 1 || move > 2)) { // If cin is not value
cout << endl << "Please enter a valid move." << endl << endl;
cout << "move: ";
cin >> move;
cin.clear();
cin.ignore(100, '\n');
}
// if move is value
enemyFound = false;
return move;
}
}
/*
void getMovement(int &player_x, int &player_y, int &chosenRow, int &chosenCol)
Takes in the current player_x and player_y as well as the user inputted new movement values.
Function prompts for user chosen new movement and sets the values of the player movement.
Return Value: None
Creator: Scott Hernandez
*/
void getMovement(int &player_x, int &player_y, int &chosenRow, int &chosenCol) {
// Get player movement
cout << "Enter next cell (row & col): ";
cin >> chosenRow >> chosenCol;
while (!cin || (abs(player_x - chosenRow) > 1) || (abs(player_y - chosenCol) > 1)) { // Loop until value is valid
cout << endl << "Please enter a valid range from player (0-1 from player)" << endl << endl;
cin.clear();
cin.ignore(100, '\n');
cout << "Enter next cell (row & col): ";
cin >> chosenRow >> chosenCol;
}
}
/*
void gameOverMessage(int& gongs)
Prints out game over message depending on gongs left
Return Value: None
Creator: Scott Hernandez
*/
void gameOverMessage(int& gongs) {
if (gongs <= 0) // If gongs are less than or equal to 0
cout << endl << "You run out of time and the fate of the tourists is forever unknown." << endl << endl;
else
cout << endl << "YOU WON THE GAME!" << endl << endl;
}
/*
int fightingPromptActions(int move, int& enemyTile, string enemyName, int& playerTile, int& player_x, int& player_y, int chosenRow, int chosenCol, int& gongs)
Returns whether player has won or lost the battle based off a random number
Return Value: 0 or 1 depending on whether the player has won(value = 1), lost(value = 0), or waited(value = 1)
Creator: Scott Hernandez
*/
int fightingPromptActions(int move, int& enemyTile, string enemyName, int& playerTile, int& player_x, int& player_y, int chosenRow, int chosenCol, int& gongs) {
int FREE = 0;
int PLAYER = 1;
switch (move) { // Switch case for fight prompt chosen values
case 1: // Waiting for enemy to leave
// Advance
cout << endl << "---> " << enemyName << " is gone ... You Advance!" << endl << endl; // Message to user
gongs -= 5; // Subtract 5 gongs
playerTile = -1; // Remove player from tile
player_x = chosenRow; // Set player x
player_y = chosenCol; // Set player y
return 1;
break;
case 2: // Fight
srand(unsigned(time(0)));
int random = rand() % 100; // Generate random number
if (random <= 49) { // If random number is equal to 0
// Advance
cout << endl << "---> You fight the " << enemyName << " and win ... You Advance!" << endl << endl; // Message to user
gongs -= 2; // Subtract 2 gongs
playerTile = -1; // Remove player from tile
player_x = chosenRow; // Set player x
player_y = chosenCol; // Set player y
return 1;
}
else {
cout << endl << "---> You fight the " << enemyName << " and lose ... Retreat!" << endl << endl;
gongs -= 3;
}
return 0;
}
}
/*
void checkGameOver(int gongs, int spaceCheck, int ENDTYPE, bool& running)
Checks for the game being over and stops the game loop if it is
Return Value: None
Creator: Scott Hernandez
*/
void checkGameOver(int gongs, int spaceCheck, int ENDTYPE, bool& running) {
if (gongs <= 0 || spaceCheck == ENDTYPE) // Check for gongs <= 0 or if the player reaches the end of the game
running = false; // End game
}
/*
setTopCoordinates()
Sets the coordinates of the top of the map
Return Value: None
Creator: Scott Hernandez
*/
void setTopCoordinates() {
for (int i = 0; i < 5; i++) // Setting up map coordinate around map with setw()
{
if (i != 0)
cout << setw(4) << i;
else
cout << setw(5) << i;
}
cout << endl;
}