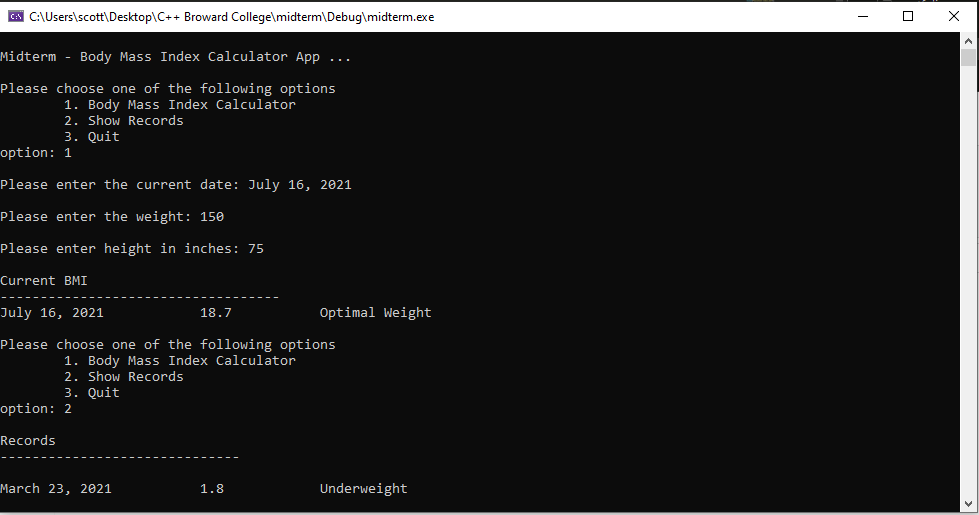
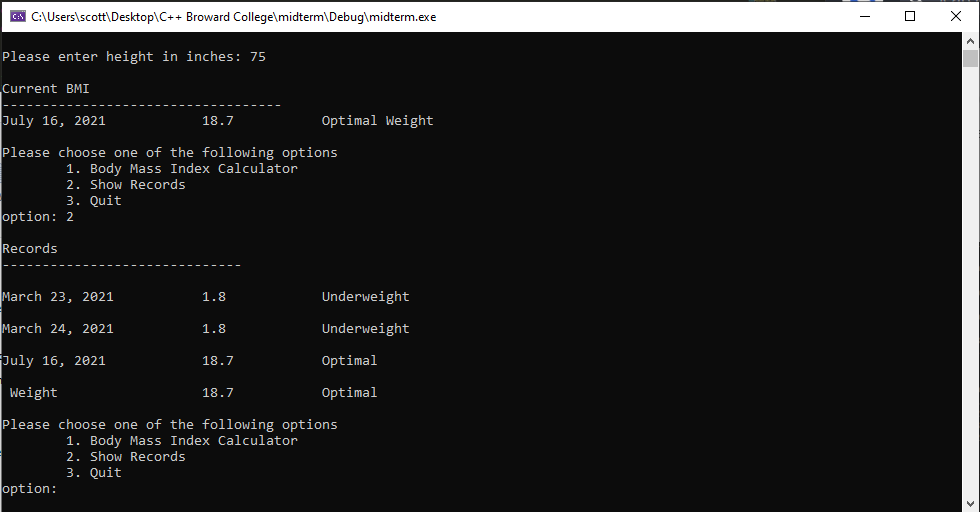
×
/*
Scott Hernandez
midterm.cpp
A body mass index calculator that displays the users BMI
Input: date, weight, height in inches
Processing: 1. Display Menu
1. Body Mass Index Calculator
Grab date, weight, height
Calculate BMI from weight and height
save to file
2. Show Records
read from file
Display records
3. Quit
Quit Program
2. Loop until option 3 is chosen
Good bye message
Output: Display menu allowing user to enter or show records
*/
// Header Files
#include <iostream>
#include <cmath>
#include <iomanip>
#include <string>
#include <fstream>
using namespace std;
int main()
{
// Variables
int option = 0;
string date, weightStanding, lineDate, lineStanding;
double weight, BMI, lineBMI;
int height;
ofstream outputFile;
ifstream inputFile;
// Start of messages
cout << endl << "Midterm - Body Mass Index Calculator App ..." << endl;
while (option != 3) { // Continue until quit
cout << endl << "Please choose one of the following options" << endl
<< "\t1. Body Mass Index Calculator" << endl // OPTION 1 - Calculator for BMI and saving to file
<< "\t2. Show Records" << endl // OPTION 2 - Show records from file
<< "\t3. Quit" << endl // OPTION 3 - QUIT
<< "option: ";
cin >> option; // Get option
while (!cin || (option < 1 || option > 3)) { // Validate for option value
cout << endl << "Invalid option! Please try again!" << endl
<< "option: ";
cin.clear();
cin.ignore(100, '\n');
cin >> option;
}
switch (option) { // switch case for options
case 1: // CASE 2
if (outputFile.is_open()) // Close file if already open
outputFile.close();
cout << endl << "Please enter the current date: "; // prompt for current date
cin.ignore();
getline(cin, date);
while (!cin || date == "") { // Continue if date is invalid
cout << endl << "Please enter a valid date" << endl;
cout << endl << "Enter current date: ";
cin.clear();
cin.ignore(100, '\n');
getline(cin, date);
}
cout << endl << "Please enter the weight: "; // prompt for weight
cin >> weight;
while (!cin || weight < 50) { // Continue if weight is invalid
cout << endl << "Please enter a weight higher than 50" << endl;
cout << endl << "Enter weight: ";
cin.clear();
cin.ignore(100, '\n');
cin >> weight;
}
cout << endl << "Please enter height in inches: "; // prompt for height
cin >> height;
while (!cin || height < 30) { // Calculate if weight is invalid
cout << endl << "Please enter a height higher than 30 inches" << endl;
cout << endl << "Enter height: ";
cin.clear();
cin.ignore(100, '\n');
cin >> height;
}
BMI = (weight * 703.0) / (pow(height, 2.0)); // Calculate BMI
if (BMI > 25) // Get weight standing
weightStanding = "Overweight";
else if (BMI > 18.5)
weightStanding = "Optimal Weight";
else
weightStanding = "Underweight";
cout << endl << "Current BMI" << endl // Display values calculated
<< "-----------------------------------" << endl
<< left << setw(25) << date << setw(15) << showpoint << fixed << setprecision(1) << BMI << setw(15) << weightStanding << endl;
outputFile.open("myBMI.txt", ios::app); // open file
outputFile << date << endl << BMI << endl << weightStanding << endl; // Save to file
outputFile.close(); // Close file
break;
case 2: // CASE 2
if (inputFile.is_open()) // Close file if open
inputFile.close();
cout << endl << "Records" << endl; // Prompt start of records
for (int i = 0; i < 30; i++) { // line of dashes with for loop
cout << "-";
}
cout << endl;
inputFile.open("myBMI.txt", ios::in); // open file with mode input
bool hasLines = false; // boolean to check if file is empty
while (getline(inputFile, lineDate)) { // Continue if file has lines
hasLines = true; // file is not empty
if (lineDate != "")
{
inputFile >> lineBMI; // get bmi from file
inputFile >> lineStanding; // get standing from file
cout << showpoint << fixed << setprecision(1);
cout << endl << left << setw(25) << lineDate << setw(15) << lineBMI << setw(15) << lineStanding << endl; // Display records
}
}
if (!hasLines) // Continue if no records
cout << endl << "No records to display!" << endl;
inputFile.close(); // Close file
break;
}
}
cout << endl << "Good Bye ..." << endl; // Good bye message
return 0;
}
March 23, 2021
1.7575
Underweight
March 24, 2021
1.7575
Underweight