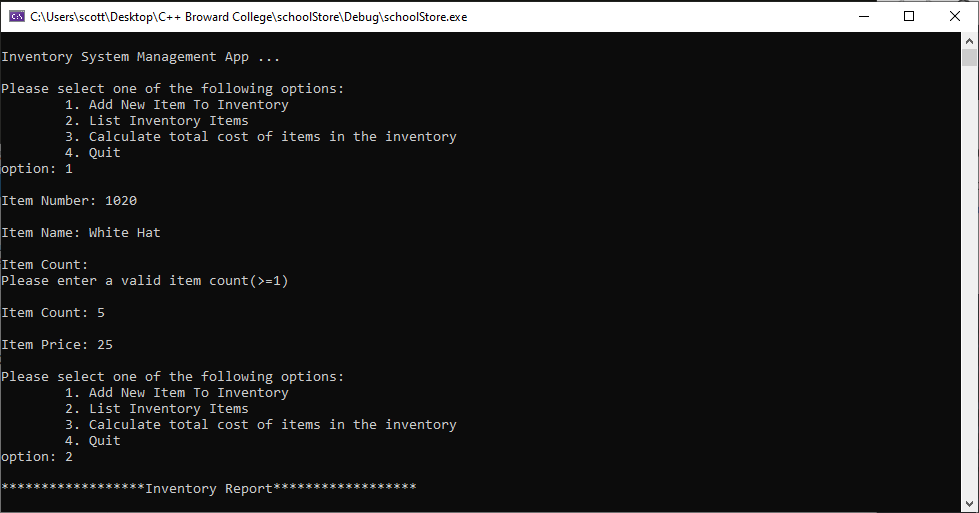
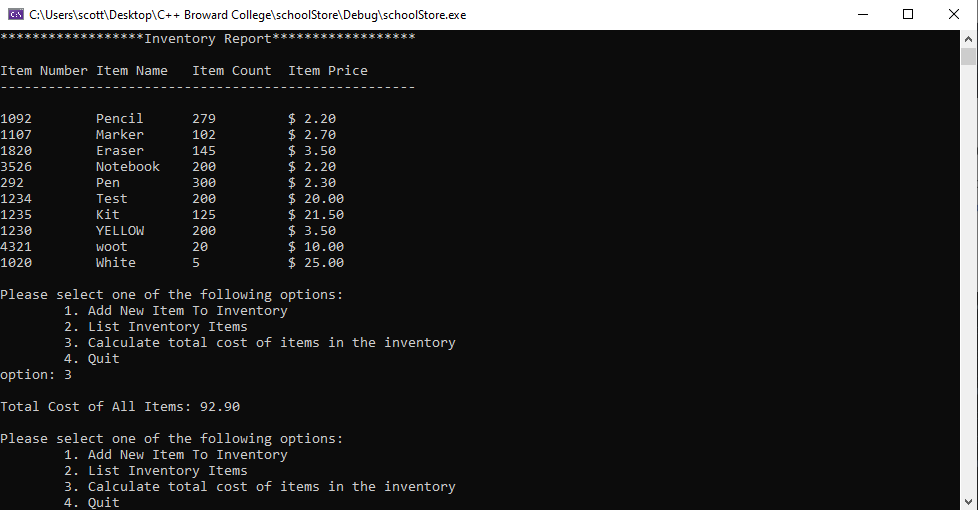
×
1092 Pencil 279 2.20
1107 Marker 102 2.70
1820 Eraser 145 3.50
3526 Notebook 200 2.20
292 Pen 300 2.3
1234 Test 200 20
1235 Kit 125 21.5
1230 YELLOW 200 3.5
4321 woot 20 10
/*
Scott Hernandez-Pauli
schoolStore.cpp
Description: An inventory system that reads inventory from a file and allows the user to view the list,
add items to it, and calculate total cost.
Input: Inventory file must be provided.
Processing: 1. Prompt user with menu that is looped
1. Add item
Add item to inventory
2. view inventory
list inventory
3. total cost
total cost of inventory
4. quit
exit program
2. Read from file
3. Create functions
readInData() // read in data
createReport() // create report of inventory
getItem() // get item to be added
addItemToFile() // add item to inventory
totalCost() // calculate total cost of items in inventory
Output: Display menu driven inventory system
*/
#include <iostream>
#include <string>
#include <fstream>
#include <iomanip>
using namespace std;
struct Inventory { // Inventory struct
int itemNumber;
string itemName;
int itemCount;
double itemPrice;
};
Inventory myInventory[50]; // Array of inventory items
Inventory newItem; // Called when new item is added
int total = 0; // Amount of items in inventory at the moment
// Prototype functions
void readInData(Inventory[], int&);
void createReport(Inventory[], int);
Inventory getItem();
void addItemToFile(Inventory);
double totalCost(Inventory[], int);
int main()
{
bool running = true; // boolean to start loop
int option = 0; // option selected by user
readInData(myInventory, total); // Read in data from file
cout << "\nInventory System Management App ...\n" << endl; // Start of program
while (running) { // Start of loop
cout << "Please select one of the following options:" << endl // Prompt user with menu
<< "\t1. Add New Item To Inventory" << endl
<< "\t2. List Inventory Items" << endl
<< "\t3. Calculate total cost of items in the inventory" << endl
<< "\t4. Quit" << endl
<< "option: ";
cin >> option;
while (!cin || option < 1 || option > 4) { // Validate option entered by user
cout << endl << "Please select another option, selected option not valid!" << endl << endl;
cout << "option: ";
cin.clear();
cin.ignore(100, '\n');
cin >> option;
}
switch (option) { // switch for menu value
case 1:
addItemToFile(getItem()); // add item to a file
break;
case 2:
readInData(myInventory, total); // read in data and create report.
createReport(myInventory, total);
break;
case 3:
cout << endl << fixed << showpoint << setprecision(2) << "Total Cost of All Items: " << totalCost(myInventory, total) << endl << endl; // Output total cost of items
break;
case 4:
cout << endl << "Good Bye ..." << endl; // Good bye message
exit(0); // end program
running = false;
break;
}
}
return 0;
}
/*
readInData(Inventory inv[], int& total)
Reads in values from file and loads them into the inventory array
Return Value: None
*/
void readInData(Inventory inv[], int& total) {
total = 0; // Amount of items
ifstream file; // input stream
file.open("inventory.txt"); // file to be opened
if (!file) { // if file does not open
cout << "Fatal Error ... Could not open inventory.txt" << endl;
exit(EXIT_FAILURE);
}
while (file >> myInventory[total].itemNumber) { // load array with items
file >> myInventory[total].itemName;
file >> myInventory[total].itemCount;
file >> myInventory[total].itemPrice;
total++;
}
file.close(); // close file
}
/*
createReport(Inventory inv[], int total)
Creates a report of all the items in the inventory
Return Value: None
*/
void createReport(Inventory inv[], int total) {
int pad = 12; // padding for the setw() functions
cout << "\n******************Inventory Report******************\n" << endl;
cout << left << setw(pad) << "Item Number" << left << setw(pad) << "Item Name" << left << setw(pad) << "Item Count" << left << setw(pad) << "Item Price" << endl;
cout << "----------------------------------------------------\n" << endl;
for (int i = 0; i < total; i++) {
cout << left << setw(pad) << inv[i].itemNumber // item number
<< left << setw(pad) << inv[i].itemName // item name
<< left << setw(pad) << inv[i].itemCount // item count
<< "$ " <<left << setw(pad) << fixed << showpoint << setprecision(2) << inv[i].itemPrice << endl; // item price
}
cout << endl;
}
/*
Inventory getItem()
Prompts the user for the item they want to add to the inventory
Return value: Item to be added
*/
Inventory getItem() {
// Prompt user for different values needed for an item
cout << endl << "Item Number: ";
cin >> newItem.itemNumber;
while (!cin) { // Validate item number
cout << endl << "Please enter a valid item number" << endl << endl
<< "Item Number: ";
cin.clear();
cin.ignore(100, '\n');
cin >> newItem.itemNumber;
}
cout << endl << "Item Name: ";
cin >> newItem.itemName;
cout << endl << "Item Count: ";
cin >> newItem.itemCount;
while (!cin || newItem.itemCount < 1) { // validate item count (>=1)
cout << endl << "Please enter a valid item count(>=1)" << endl << endl
<< "Item Count: ";
cin.clear();
cin.ignore(100, '\n');
cin >> newItem.itemCount;
}
cout << endl << "Item Price: ";
cin >> newItem.itemPrice;
while (!cin || newItem.itemPrice < 1) { // Validate item price(>= 1)
cout << endl << "Please enter a valid item price(>=1)" << endl << endl
<< "Item Price: ";
cin.clear();
cin.ignore(100, '\n');
cin >> newItem.itemPrice;
}
cout << endl;
return newItem; // Return item to be added
}
/*
addItemToFile(Inventory item)
Add item supplied to the inventory
Return Value: None
*/
void addItemToFile(Inventory item) {
ofstream file; // output stream
file.open("inventory.txt", ios::app); // open file and append
if (!file) { // if file does not exist
cout << "Fatal Error ... Could not open inventory.txt. Failed to create Item!" << endl;
}
else {
file << item.itemNumber << " " << item.itemName << " " << item.itemCount << " " << item.itemPrice << endl; // Add item to file
}
}
/*
totalCost(Inventory inv[], int total)
Calculate total cost of inventory
Return Value: total value of inventory
*/
double totalCost(Inventory inv[], int total) {
double amount = 0; // cost of inventory
for (int i = 0; i < total; i++) { // loop through each item and add them up
amount += inv[i].itemPrice;
}
return amount; // return amount
}