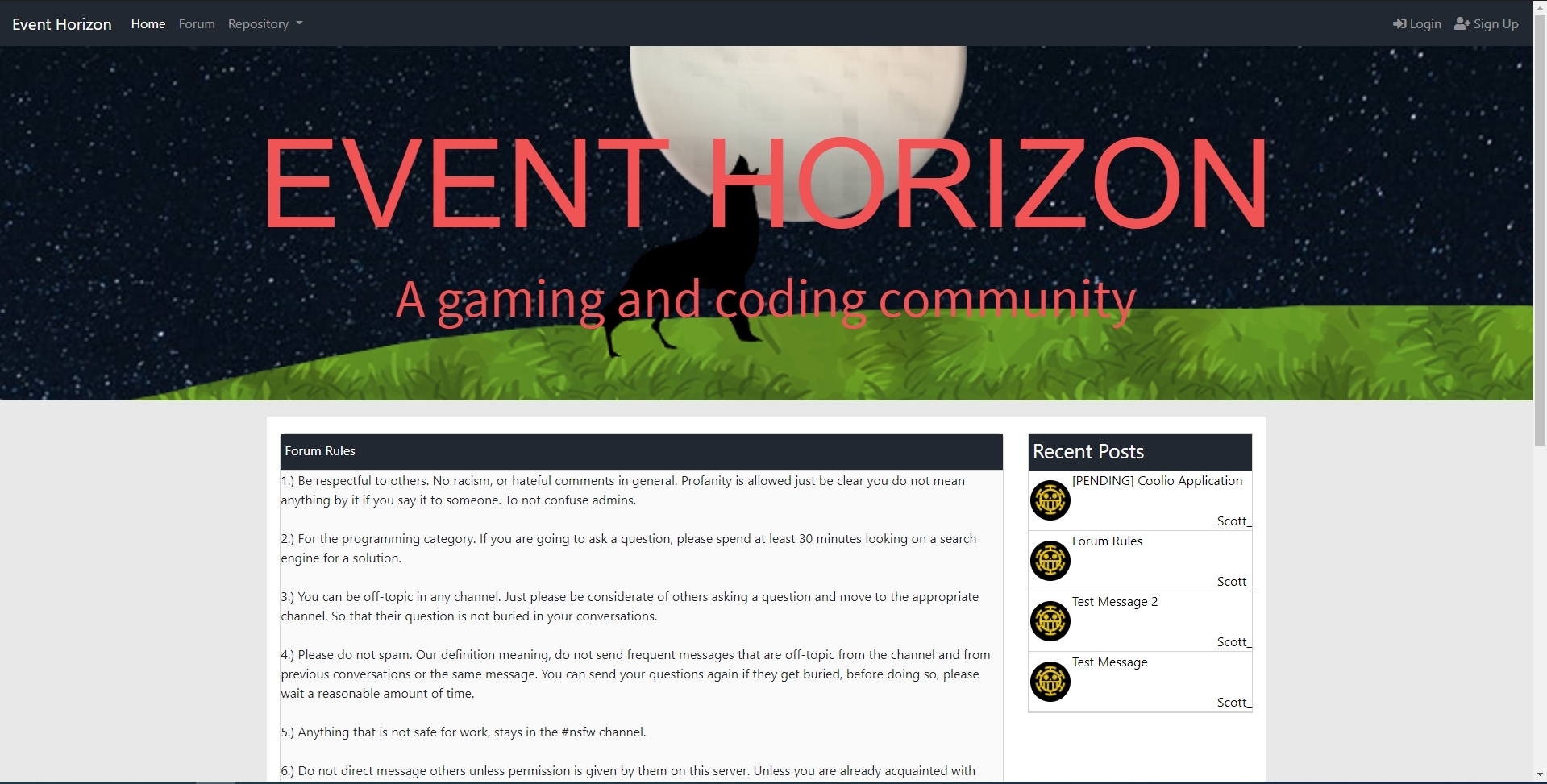
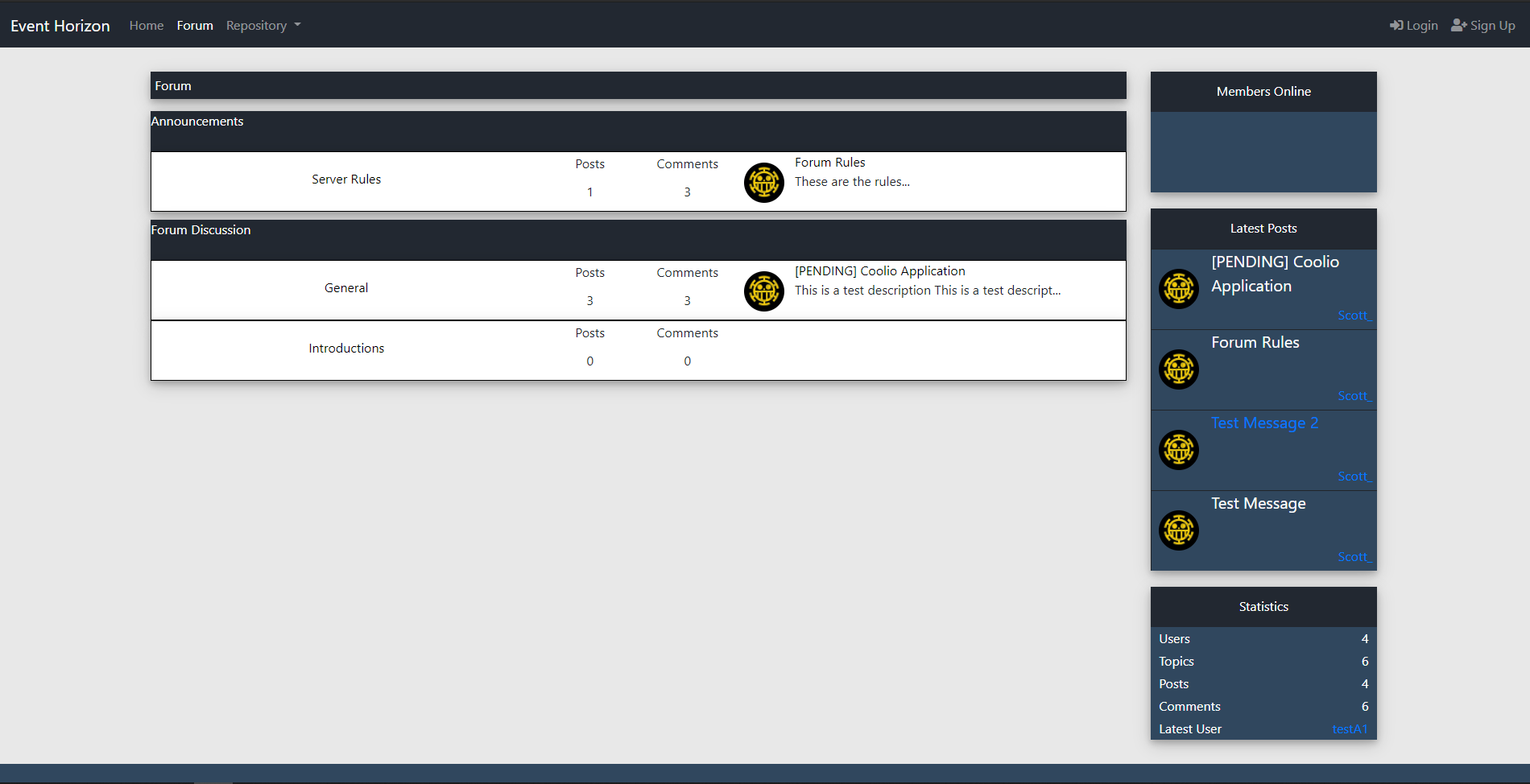
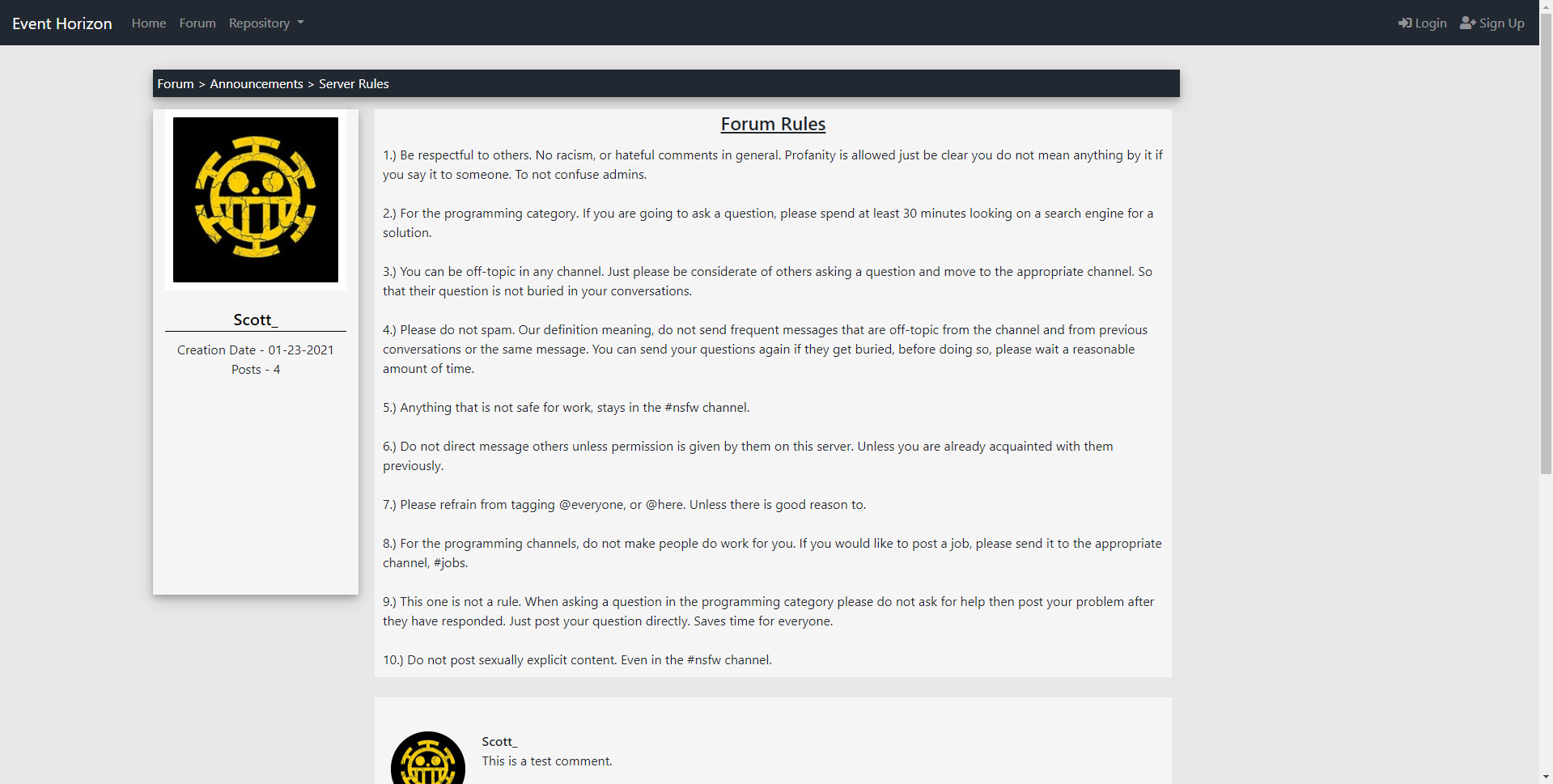
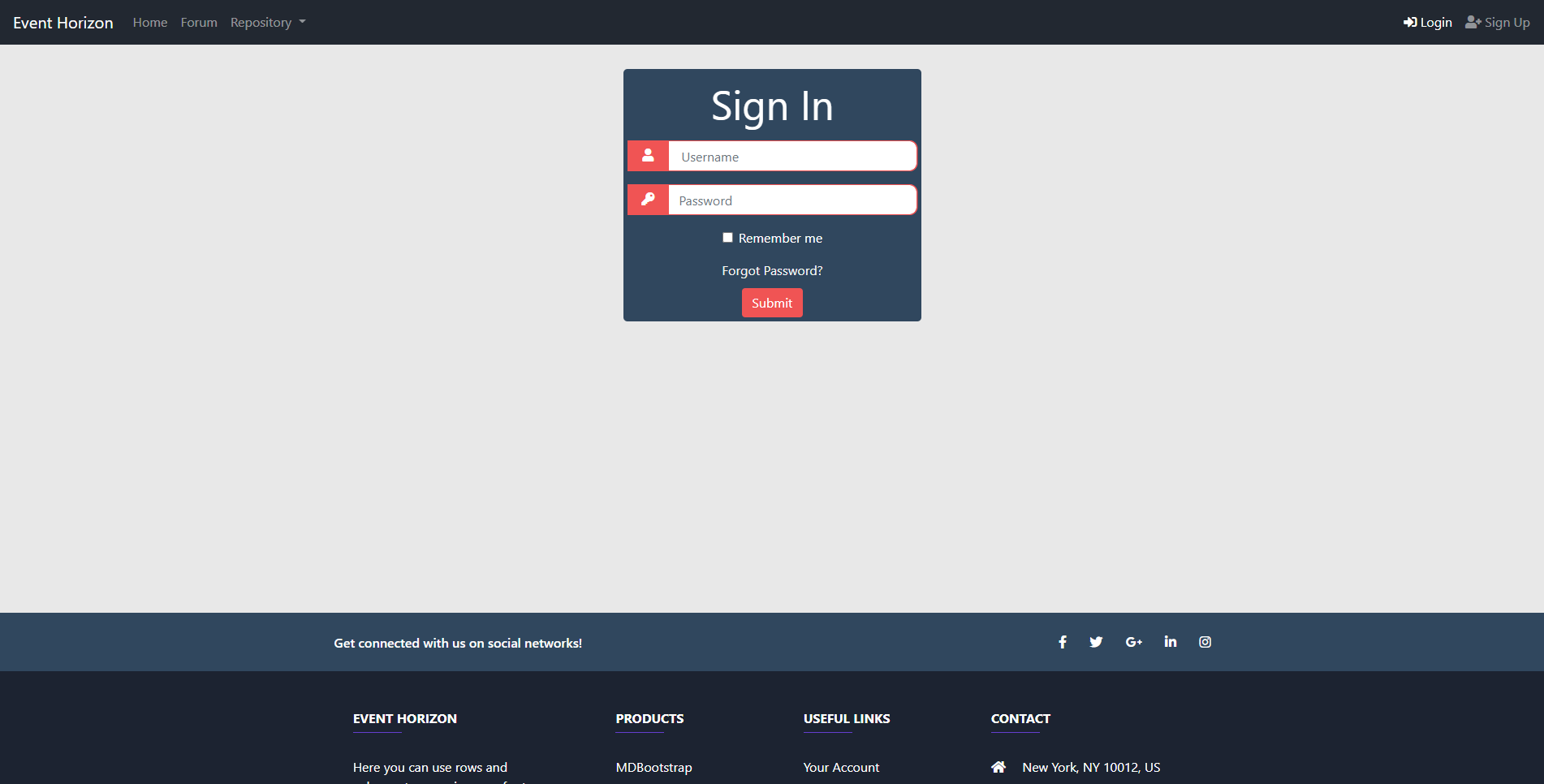
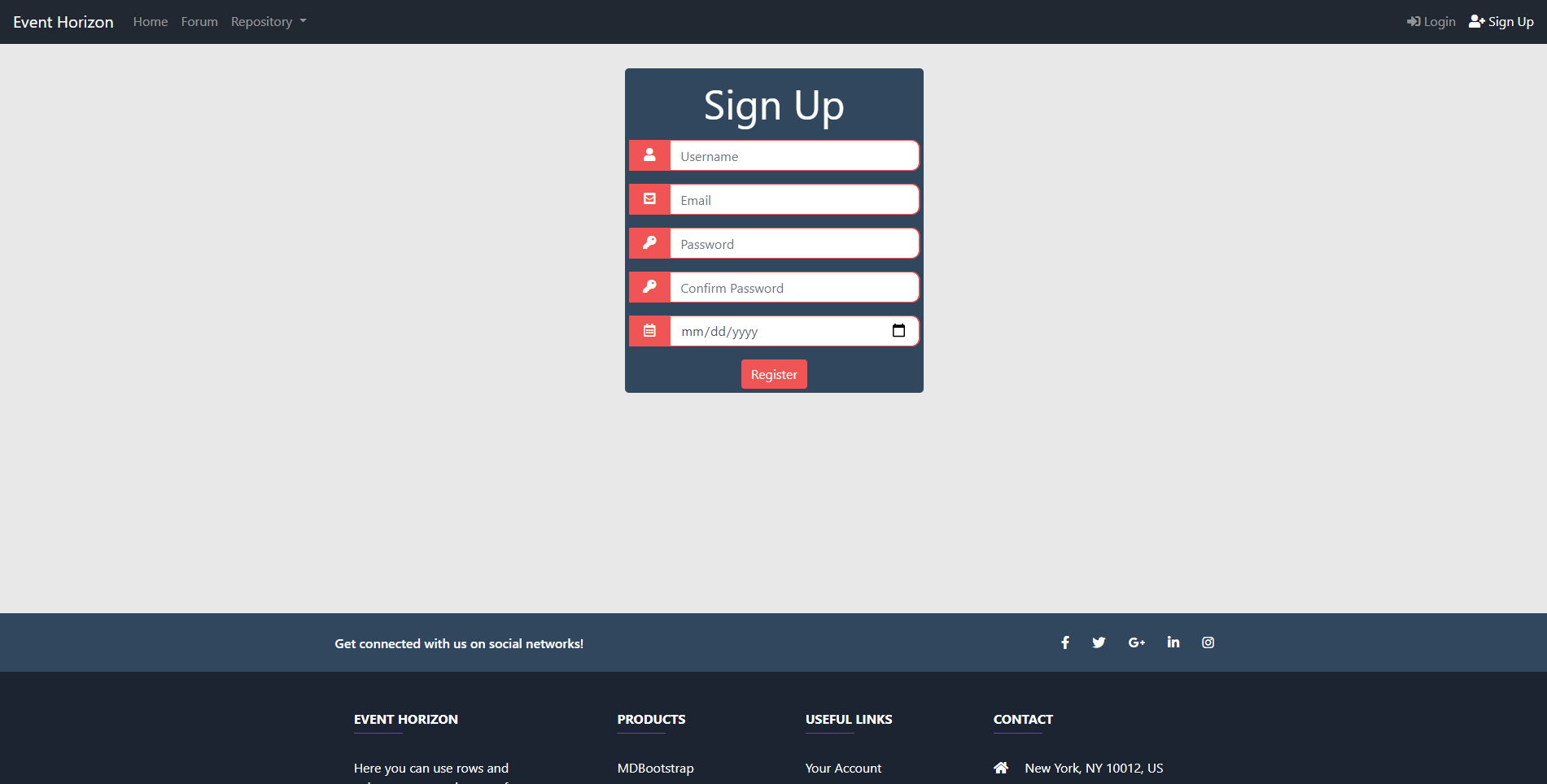
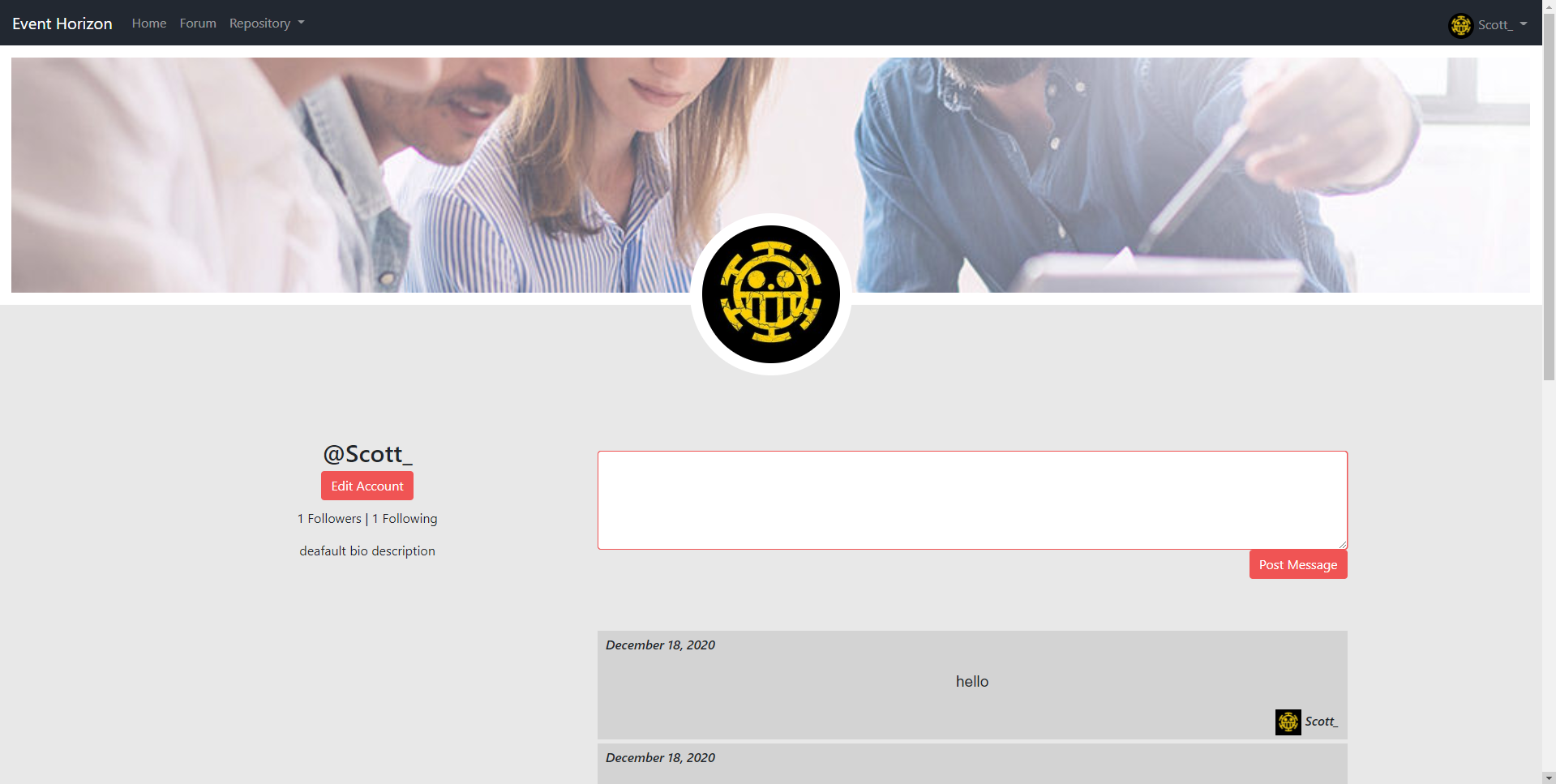
×
<?php
require 'header.php';
require 'libraries/Forum.php';
if (!isset($_GET['post'])) {
header('Location: forum.php?');
die();
}
$post = $_GET['post'];
if (Forum::getPost($post) == NULL) {
header('Location: forum.php?message='.$post);
die();
}
$post = Forum::getPost($post);
$userid = $post['user_id'];
$username = Account::getData(Account::USERNAME, $userid);
$avatar = Account::getData(Account::AVATAR, $userid);
$dt = new DateTime($post['creation_date']);
$dt = $dt->format('m-d-Y');
$userPostCount = Account::getUserForumPosts($userid);
if (isset($_POST['postcomment'])) {
$m = nl2br($_POST['posttextarea']);
$p = preg_replace('~[\r\n]+~', '', $m);;
$message = htmlentities($p);
$timestamp = date('Y-m-d h-m-s');
$replyvalue = 0;
if (isset($_POST['replyvalue']))
$replyvalue = $_POST['replyvalue'];
Forum::createComment(Account::getData(Account::ID), $post['id'], $timestamp, $message, $replyvalue);
}
// action tabs
if (isset($_POST['addtohomepage'])) {
Forum::setHomepage($post['id']);
}
?>
<div class="content">
<div class="ThreadPost">
<div class="row">
<div class="col-md-10 offset-md-1">
<div class="breadcrumbs col-md-10">
<?php print_r(Forum::breadCrumbs(Forum::getTopicNameFromID($post['topic_id']), true)); ?>
</div>
<div id="border">
<div id="messageboard">
<div class="row">
<div class="col-md-2" id="userinfo">
<img id="avatar" src="<?php echo $avatar; ?>" />
<!--<a id="username" href="<?php echo 'profile.php?profile=' . $username;?>"><?php echo $username; ?></a>-->
<p id="username"><a href="<?php echo 'profile.php?profile=' . $username; ?>"><?php echo $username; ?> </a></p>
<div id="divider"></div>
<p id="creationdate">Creation Date - <?php echo $dt; ?></p>
<p id="userposts">Posts - <?php echo $userPostCount; ?></p>
</div>
<div class="col-md-8" id="message">
<?php if (Account::isLoggedIn()) { ?>
<div id="actiontab">
<div id="borderab">
<form action="" method="POST">
<?php
if (Forum::getHomepage($post['id']) == Forum::HOMEPAGE_ACTIVE)
echo '<input id="removefromhomepage" type="submit" name="addtohomepage" value="" title="Click here to remove from home page">';
else
echo '<input id="addtohomepage" type="submit" name="addtohomepage" value="" title="Click here to add to home page">';
?>
<input id="editpost" type="submit" name="editpost" value="" title="Click here to edit your post">
<input id="deletepost" type="submit" name="deletepost" value="" title="Click here to delete this post">
</form>
</div>
</div>
<?php } ?>
<div id="header">
<div id="title"><?php echo $post['title']; ?></div>
</div>
<div id="content">
<?php
$message = html_entity_decode($post['message']);
$message = str_replace('"', '\"', $message);
$message = str_replace('\'', '\"', $message);
echo nl2br($message);
?>
</div>
<div class="threadcomments">
<div id="commentbox">
<?php if (Account::isLoggedIn()) { ?>
<div id="replybox"></div>
<form action="" method="POST">
<textarea name="posttextarea" rows="4" placeholder="Enter your comment here..."></textarea>
<input class="btn btn-primary" type="submit" name="postcomment">
</form>
<?php } ?>
</div>
<div id="usercomments">
<?php
$comments = Forum::getPostComments($post['id']);
if (isset($comments[0]['message'])) {
foreach ($comments as $c) {
$avatar = Account::getData(Account::AVATAR, $c['user_id']);
$username = Account::getData(Account::USERNAME, $c['user_id']);
$message = html_entity_decode($c['message']);
$message = str_replace('"', '\"', $message);
$message = str_replace('\'', '\"', $message);
?>
<div id="comments">
<div id="avatar"><img src="<?php echo $avatar; ?>" /></div>
<div id="messagebox">
<div id="name"><?php echo $username; ?></div>
<div id="message"><?php echo nl2br($message); ?></div>
</div>
<?php if (Account::isLoggedIn()) { ?>
<div id="reply">
<button data-id="<?php echo $c['id'] . '|' . $username; ?>" class="btn btn-primary" name="replysubmit" >reply</button>
</div>
<?php } ?>
</div>
<?php
}
} else {
echo 'No comments.';
}
?>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
<script>
$('.threadcomments #usercomments #comments #reply button').click(function() {
var string = $(this).attr('data-id');
var result = string.split('|');
var tableid = result[0];
var name = result[1];
$('.threadcomments #commentbox #replybox').html('<div id="replytab">'+name+" x</div>");
if ($('.threadcomments #commentbox form').has('#replyvalue')) {
$('.threadcomments #commentbox form #replyvalue').remove();
}
$('.threadcomments #commentbox form').append('<input id="replyvalue" type="hidden" name="replyvalue" value="'+tableid+'">');
});
$('.threadcomments #commentbox #replybox').click(function(e) {
if (e.target.id == 'replytab') {
$(this).children().remove();
if ($('.threadcomments #commentbox form').has('#replyvalue')) {
$('.threadcomments #commentbox form #replyvalue').remove();
}
}
});
</script>
<?php
require 'footer.php';
?>
<?php
require 'libraries/cookies.php';
require 'libraries/Account.php';
require 'libraries/Forum.php';
if (isset($_GET['code'])) {
if ($_GET['code'] == 'signout') {
Account::signOut();
header('Location: index.php');
die();
}
}
if (isset($_POST['createforumpostcreate'])) {
$topic = $_POST['topicName'];
$title = $_POST['title'];
$description = $_POST['description'];
$m = nl2br($_POST['post']);
$p = preg_replace('~[\r\n]+~', '', $m);;
$post = htmlentities($p);
$timestamp = date('Y-m-d h-m-s');
Forum::createPost(Account::getData(Account::ID),
Forum::getTopicIDFromName($topic),
$timestamp,
$title,
$description,
$post);
header('Location: forum.php?topic='.$topic);
die();
}
/*
posts
id
user_id
topic_id
creation_date
title
description
message
*/
if (isset($_POST['createforumpostcancel'])) {
$topic = $_POST['topicName'];
header('Location: forum.php?topic='.$_POST['topicName']);
die();
}
if (isset($_POST['follow'])) {
$userfollowed = $_POST['user'];
$id = Account::getIDFromType(Account::USERNAME, $userfollowed);
Account::followUser($id);
header('Location: profile.php?profile='.$userfollowed);
die();
}
if (isset($_POST['block'])) {
$userfollowed = $_POST['user'];
$id = Account::getIDFromType(Account::USERNAME, $userfollowed);
Account::blockUser($id);
header('Location: profile.php?profile='.$userfollowed);
die();
}
if (isset($_POST['loginaccount'])) {
DB::createLoginTokensTable();
$username = $_POST['username'];
$password = $_POST['password'];
$rememberme = '';
try {
if (!isset($_POST['rememberme'])) $rememberme = false; else $rememberme = true;
} catch(Exception $e) {$rememberme = false;}
if (!empty($username) || !empty($password)) {
$val = Account::verify($username, $password);
if (Account::verify($username, $password)) {
// account verified
if($rememberme)
cookies::setCookie('remembermeusername', $username, cookies::TIME_MONTH);
else
cookies::removeCookie('remembermeusername');
$user_id = Account::getIDFromType(Account::USERNAME, $username);
Account::createLoginCookies($user_id);
header('Location: index.php');
die();
}
}
cookies::removeCookie('remembermeusername');
LoginErrorMessage('Account credentials incorrect or account does not exist!' . $val);
}
if (isset($_POST['register'])) {
DB::createUserTable();
$email = $_POST['email'];
$username = $_POST['username'];
$password = $_POST['password'];
$confirmpassword = $_POST['confirmpassword'];
$birthdate = $_POST['birthdate'];
if (!empty($email) && !empty($username) && !empty($password) && !empty($confirmpassword) && !empty($birthdate)) {
// Fields are not empty
if (strlen($username) >= 6 && strlen($username) <= 32) {
// enough characters
if (preg_match('/[a-zA-Z0-9_]+/', $username)) {
// Username matches regex.
if (DB::getData('accounts', 'username', 'username', $username) == NULL) {
// Username does not exist. Continue.
if (filter_var($email, FILTER_VALIDATE_EMAIL)) {
// Email is valid
if (DB::getData('accounts', 'email', 'username', $username) == NULL) {
// Email does not exist! Continue.
if (strlen($password) >= 6 && strlen($password) <= 60) {
// password within size.
$upper = preg_match('@[A-Z]@', $password);
$lower = preg_match('@[a-z]@', $password);
$number = preg_match('@[0-9]@', $password);
$special = preg_match('@[^\w]@', $password);
if ($upper && $lower && $number && $special) {
// Password is strong. Continue.
if ($password == $confirmpassword) {
// Passwords match. Continue.
if (userAge($birthdate) >= 13) {
// User is old enough. Continue.
$timestamp = date('Y-m-d h-m-s');
$hash = password_hash($password, PASSWORD_BCRYPT);
DB::setData('accounts', array('RegistrationDate'=>$timestamp, 'Username'=>$username, 'Password'=>$hash, 'Email'=>$email, 'Role'=>'member', 'BirthDate'=>$birthdate, 'Verified'=>'0', 'ProfileImg'=>''));
// ACCOUNT CREATED AND LOGGED IN
$user_id = Account::getIDFromType(Account::USERNAME, $username);
Account::createLoginCookies($user_id);
header('Location: index.php');
die();
} else {
RegisterErrorMessage('You are too young to make an account with us!');
}
} else {
RegisterErrorMessage('Passwords do not match!');
}
} else {
RegisterErrorMessage('Password must have an uppercase letter, a lowercase letter, a special character, and be greater than 8 characters!');
}
} else {
RegisterErrorMessage('Too little or too many characters for a password!');
}
} else {
RegisterErrorMessage('Email already exists!');
}
} else {
RegisterErrorMessage('Email is not valid!');
}
} else {
RegisterErrorMessage('This username already exist!');
}
} else {
RegisterErrorMessage('Username may only be alphanumeric!');
}
} else {
RegisterErrorMessage('Too little or too many characters for a username!');
}
} else {
RegisterErrorMessage('One or multiple fields empty!');
}
}
function RegisterErrorMessage($errmessage) {
$username = urlencode($_POST['username']);
$email = $_POST['email'];
$birthdate = $_POST['birthdate'];
$message = 'enc=true&username='.$username.'&email='.$email.'&birthdate='.$birthdate;
$message = $message . '&errormessage=' . $errmessage;
header('Location: signup.php?'.urlencode($message));
}
function LoginErrorMessage($message) {
$m = 'enc=true&errormessage='.$message;
header('Location: login.php?'.$m);
}
function passwordStrength($password) {
$upper = preg_match('@[A-Z]@', $password);
$lower = preg_match('@[a-z]@', $password);
$number = preg_match('@[0-9]@', $password);
$special = preg_match('@[^\w]@', $password);
if (!$upper || !$lower || !$number || !$special || strlen($password) < 8)
return true;
return false;
}
function userAge($date) {
$year = explode('-', $date);
$age = date('Y') - $year[0];
if (date('md') < date('md', strtotime($date))) {
return $age - 1;
}
return $age;
}
<?php require 'header.php';
require 'libraries/Forum.php';
if (isset($_POST['catnamesubmit'])) {
$name = $_POST['catname'];
if ($name == NULL || trim($name) == '')
return;
Forum::createCategory($name);
}
if (isset($_POST['topicnamesubmit'])) {
$catValue = $_POST['topicselectvalue']; // ID VALUE
$topicname = $_POST['topicname'];
if (strpos($catValue, '^') !== FALSE) { // IS TOPIC
$topicValue = str_replace('^', '', $catValue);
echo 'IS TOPIC ' . $catValue;
$categoryID = Forum::getCategoryFromTopic($topicValue);
Forum::createTopic($categoryID, $topicname, '', $topicValue);
} else { // IS CATEGORY
echo 'IS CATEGORY ' . $catValue;
Forum::createTopic($catValue, $topicname, '');
}
/*
$topicname = $_POST['topicname'];
if ($catValue != 'none' && trim($topicname) != '') {
Forum::createTopic($catValue, $topicname, '');
}
*/
}
?>
<div class="content">
<h2>Admin Panel</h2>
<!-- Nav tabs -->
<ul class="nav nav-tabs" id="myTab" role="tablist">
<li class="nav-item">
<a class="nav-link active" id="ranks-tab" data-toggle="tab" href="#ranks" role="tab" aria-controls="ranks" aria-selected="true">Ranks</a>
</li>
<li class="nav-item">
<a class="nav-link" id="forum-tab" data-toggle="tab" href="#forum" role="tab" aria-controls="forum" aria-selected="false">Forum</a>
</li>
<li class="nav-item">
<a class="nav-link" id="messages-tab" data-toggle="tab" href="#messages" role="tab" aria-controls="messages" aria-selected="false">Messages</a>
</li>
<li class="nav-item">
<a class="nav-link" id="settings-tab" data-toggle="tab" href="#settings" role="tab" aria-controls="settings" aria-selected="false">Settings</a>
</li>
</ul>
<!-- Tab panes -->
<div class="tab-content">
<div class="tab-pane active" id="ranks" role="tabpanel" aria-labelledby="ranks-tab">
Ranks Stuff
</div>
<div class="tab-pane" id="forum" role="tabpanel" aria-labelledby="forum-tab">
Forum Stuff
<form action="" method="POST">
<input type="text" id="catname" name="catname"><br>
<input type="submit" name="catnamesubmit" value="submit">
</form>
<br>
<form action="" method="POST">
<select name="topicselectvalue">
<option value="none">None Selected</option>
<?php
//$parents = Forum::getCategoryParents();
foreach (Forum::getCategories() as $cat) {
echo '<option value="'.$cat['id'].'">'.$cat['name'].'</option>';
allOptions($cat['id']);
}
function allOptions($catID) {
$topics = Forum::getAllTopicsInCategory($catID);
if ($topics[0]['name'] == NULL) return;
foreach ($topics as $t) {
echo '<option value="^'.$t['id'].'">> '.$t['name'].'</option>';
if ($t != NULL)
allOptions($t);
}
}
?>
</select>
<input type="text" id="topicname" name="topicname"><br>
<input type="submit" name="topicnamesubmit" value="submit">
</form>
</div>
<div class="tab-pane" id="messages" role="tabpanel" aria-labelledby="messages-tab">...</div>
<div class="tab-pane" id="settings" role="tabpanel" aria-labelledby="settings-tab">...</div>
</div>
</div>
<!--
<script>
var info = {};
info['currentPostID'] = <?=json_encode($latestPostID);?>;
function check() {
$.ajax({
type: 'POST',
url: 'c.php',
dataType: 'json',
data: info,
success: function(data){
info['currentPostID'] = parseFloat(data.latestID);
console.log(info['currentPostID']);
}
});
}
setInterval(check, 1000);
</script>
-->
<?php require 'footer.php'; ?>
<?php
$errors = array(); //To store errors
$form_data = array(); //Pass back the data to `form.php`
if (isset($_POST['currentPostID'])) {
$latestID = $_POST['currentPostID'];
$form_data['latestID'] = $latestID;
}
//Return the data back to form.php
echo json_encode($form_data);
<?php
require 'header.php';
if (isset($_POST['PostThread'])) {
echo 'TOPIC ';
echo ' : '. $_POST['topicName'];
} else {
header('Location: forum.php');
die();
}
?>
<div class="content" >
<div class="createpost">
<div class="row">
<div class="col-md-8 offset-md-2">
<h1>Create post</h1>
<form action="action.php" method="POST">
<input type="hidden" name="topicName" value="<?php echo $_POST['topicName']; ?>">
<div class="form-group">
<label for="title">Title <span class="require">*</span></label>
<input type="text" class="form-control" name="title" />
</div>
<div class="form-group">
<label for="description">Description</label>
<input type="text" class="form-control" name="description" />
</div>
<div class="form-group">
<label for="description">Post</label>
<textarea rows="7" class="form-control" name="post" ></textarea>
</div>
<div class="form-group">
<p><span class="require">*</span> - required fields</p>
</div>
<div class="form-group">
<button type="submit" class="btn btn-primary" name="createforumpostcreate">
Create
</button>
<button class="btn btn-default" name="createforumpostcancel">
Cancel
</button>
</div>
</form>
</div>
</div>
</div>
</div>
<?php
require 'footer.php';
?>
css
>
<?php
include 'header.php';
include 'libraries/Image.php';
if (!Account::isLoggedIn()) {
header('Location: index.php');
die();
}
$id = Account::getData(Account::ID);
echo $id;
if (isset($_POST['submitAvatar']))
Image::uploadImage('avatarupload', 'UPDATE accounts SET profileimg=:avatarupload WHERE id=:userid', array(':userid'=>$id));
if (isset($_POST['submitBanner']))
//Image::uploadImage('bannerupload', 'UPDATE users SET profileimg=:bannerupload WHERE id=:userid', array(':userid'=>$id)); // create database for profile
?>
<style>.content { padding: 0px; }</style>
<!-- CONTENT -->
<div class="content">
<div class="profile-editform">
<div id="avatar">
<form action="" method="post" enctype="multipart/form-data">
<label for="avatarlabel">Set your avatar image here</label>
<input type="file" name="avatarupload" id="avatarupload" accept='image/jpeg'>
<input class="btn btn-primary" type="submit" value="Upload Image" name="submitAvatar">
</form>
</div>
<hr>
<div id="banner">
<form action="" method="post" enctype="multipart/form-data">
<label for="bannerlabel">Set your banner image here</label>
<input type="file" name="bannerUpload" id="bannerupload" accept='image/jpeg'>
<input class="btn btn-primary" type="submit" value="Upload Image" name="submitBanner">
</form>
</div>
<hr>
<div id="biography">
<form class="form-group">
<label for="biolabel">Enter your biography here</label>
<textarea class="form-control" name="message" rows="3" >The cat was playing in the garden.</textarea>
<input class="btn btn-primary" type="submit" value="Set Biography" name="submitBio">
</form>
</div>
</div>
</div>
<!-- -->
<?php include('footer.php'); ?>
</div>
<!-- Footer -->
<footer class="page-footer font-small unique-color-dark" style="background-color: rgb(28, 35, 49);">
<div style="background-color: #30475e;">
<div class="container">
<!-- Grid row-->
<div class="row py-4 d-flex align-items-center">
<!-- Grid column -->
<div class="col-md-6 col-lg-5 text-center text-md-left mb-4 mb-md-0">
<h6 class="mb-0">Get connected with us on social networks!</h6>
</div>
<!-- Grid column -->
<!-- Grid column -->
<div class="col-md-6 col-lg-7 text-center text-md-right">
<!-- Facebook -->
<a class="fb-ic">
<i class="fab fa-facebook-f white-text mr-4"> </i>
</a>
<!-- Twitter -->
<a class="tw-ic">
<i class="fab fa-twitter white-text mr-4"> </i>
</a>
<!-- Google +-->
<a class="gplus-ic">
<i class="fab fa-google-plus-g white-text mr-4"> </i>
</a>
<!--Linkedin -->
<a class="li-ic">
<i class="fab fa-linkedin-in white-text mr-4"> </i>
</a>
<!--Instagram-->
<a class="ins-ic">
<i class="fab fa-instagram white-text"> </i>
</a>
</div>
<!-- Grid column -->
</div>
<!-- Grid row-->
</div>
</div>
<!-- Footer Links -->
<div class="container text-center text-md-left mt-5">
<!-- Grid row -->
<div class="row mt-3">
<!-- Grid column -->
<div class="col-md-3 col-lg-4 col-xl-3 mx-auto mb-4">
<!-- Content -->
<h6 class="text-uppercase font-weight-bold">Event Horizon</h6>
<hr class="deep-purple accent-2 mb-4 mt-0 d-inline-block mx-auto" style="width: 60px;">
<p>Here you can use rows and columns to organize your footer content. Lorem ipsum dolor sit amet,
consectetur
adipisicing elit.</p>
</div>
<!-- Grid column -->
<!-- Grid column -->
<div class="col-md-2 col-lg-2 col-xl-2 mx-auto mb-4">
<!-- Links -->
<h6 class="text-uppercase font-weight-bold">Products</h6>
<hr class="deep-purple accent-2 mb-4 mt-0 d-inline-block mx-auto" style="width: 60px;">
<p>
<a href="#!">MDBootstrap</a>
</p>
<p>
<a href="#!">MDWordPress</a>
</p>
<p>
<a href="#!">BrandFlow</a>
</p>
<p>
<a href="#!">Bootstrap Angular</a>
</p>
</div>
<!-- Grid column -->
<!-- Grid column -->
<div class="col-md-3 col-lg-2 col-xl-2 mx-auto mb-4">
<!-- Links -->
<h6 class="text-uppercase font-weight-bold">Useful links</h6>
<hr class="deep-purple accent-2 mb-4 mt-0 d-inline-block mx-auto" style="width: 60px;">
<p>
<a href="#!">Your Account</a>
</p>
<p>
<a href="#!">Become an Affiliate</a>
</p>
<p>
<a href="#!">Shipping Rates</a>
</p>
<p>
<a href="#!">Help</a>
</p>
</div>
<!-- Grid column -->
<!-- Grid column -->
<div class="col-md-4 col-lg-3 col-xl-3 mx-auto mb-md-0 mb-4">
<!-- Links -->
<h6 class="text-uppercase font-weight-bold">Contact</h6>
<hr class="deep-purple accent-2 mb-4 mt-0 d-inline-block mx-auto" style="width: 60px;">
<p>
<i class="fas fa-home mr-3"></i> New York, NY 10012, US</p>
<p>
<i class="fas fa-envelope mr-3"></i> info@example.com</p>
<p>
<i class="fas fa-phone mr-3"></i> + 01 234 567 88</p>
<p>
<i class="fas fa-print mr-3"></i> + 01 234 567 89</p>
</div>
<!-- Grid column -->
</div>
<!-- Grid row -->
</div>
<!-- Footer Links -->
<!-- Copyright -->
<div class="footer-copyright text-center py-3" style="color: gray;">© 2020 Copyright:
<a href="#"> ComplexRP.com</a>
</div>
<!-- Copyright -->
</footer>
<!-- Footer -->
<script
src="https://code.jquery.com/jquery-3.4.1.min.js"
integrity="sha256-CSXorXvZcTkaix6Yvo6HppcZGetbYMGWSFlBw8HfCJo="
crossorigin="anonymous"></script>
<script type="text/javascript" src="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/js/bootstrap.min.js"></script>
</body>
</html>
<?php require 'header.php';
DB::createForumCategories();
DB::createForumTopics();
DB::createForumPosts();
DB::createForumComments();
require 'libraries/Forum.php';
$cats = Forum::getCategories();
?>
<div class="content">
<div class="row">
<div class="col-md-8 offset-md-1">
<div class="forum">
<div class="breadcrumbs">
<?php
if (isset($_GET['topic'])) {
print_r(Forum::breadCrumbs($_GET['topic']));
if (Account::IsLoggedIn()) {
?>
<form action="createforumpost.php" method="POST">
<input type="hidden" id="" name="topicName" value="<?php echo $_GET['topic']; ?>">
<input class="btn btn-primary" type="submit" value="Post" name="PostThread">
</form>
<?php
}
} else {
print_r(Forum::breadCrumbs());
}
?>
</div>
<?php
if (!isset($_GET['topic'])) {
if (isset($_GET['category'])) {
$cat = Forum::getCategory($_GET['category']);
if ($cat != NULL)
echo '<div id="category">'.$cat['name'].'</div>';
$topics = Forum::getTopicsInCategory($cat['id']);
if ($topics != NULL) {
for ($i = 0; $i < count($topics); $i++) {
echo Forum::getTopicEcho($topics[$i]);
}
}
} else {
if ($cats != NULL)
foreach ($cats as $cat) {
echo '<div id="category">'.$cat['name'].'</div>';
$topics = Forum::getTopicsInCategory($cat['id']);
if ($topics != NULL) {
for ($i = 0; $i < count($topics); $i++) {
echo Forum::getTopicEcho($topics[$i]);
}
}
}
}
} else {
$topicname = $_GET['topic'];
$topic_id = Forum::getTopicIDFromName($topicname);
$category_id = Forum::getCategoryFromTopic($topic_id);
$topics = Forum::getTopicsInCategory($category_id, $topic_id);
if ($topics != NULL) {
for ($i = 0; $i < count($topics); $i++) {
if (isset($topics[$i]['name']))
echo Forum::getTopicEcho($topics[$i]);
}
}
$topicname = $_GET['topic'];
$topic_id = Forum::getTopicIDFromName($topicname);
$posts = Forum::getPosts($topic_id);
if ($posts[0]['title'] != NULL) {
for ($i = 0; $i < count($posts); $i++) {
echo '<div class="topicpost">';
echo '<div id="post"><a href="Thread.php?post='.$posts[$i]['id'].'">'.$posts[$i]['title'].'</a></div>';
echo '<div id="description">'.$posts[$i]['description'].'</div>';
echo '</div>';
}
} else {
if (Forum::getTopicIDFromName($_GET['topic']) != NULL) {
echo 'no posts';
} else {
header('Location: forum.php');
die();
}
}
}
?>
</div>
</div>
<div class="col-md-2 sidebar">
<div id="membersonline">
<div id="header">
<p>Members Online</p>
</div>
<div id="content"></div>
</div>
<div id="latestposts">
<div id="header">
<p>Latest Posts</p>
</div>
<div id="content">
<?php
$limit = 10;
foreach(Forum::getForumLatestPost($limit) as $p) {
$username = Account::getData(Account::USERNAME, $p['user_id']);
?>
<div id="post">
<div id="avatarimage"><a href="profile.php?profile=<?php echo $username; ?>"><img src="<?php echo Account::getData(Account::AVATAR, $p['user_id']); ?>" /></a></div>
<div id="infoDiv">
<div id="title"><a href="Thread.php?post=<?php echo $p['id']; ?>"><?php echo $p['title']; ?></a></div>
<div id="name"><a href="profile.php?profile=<?php echo $username; ?>"><?php echo $username; ?></a></div>
</div>
</div>
<?php
}
?>
</div>
</div>
<div id="statistics">
<div id="header">
<p>Statistics</p>
</div>
<div id="content">
<div id="users">
<div id="info">Users</div>
<div id="value"><?php echo Account::getUserCount(); ?></div>
</div>
<div id="topics">
<div id="info">Topics</div>
<div id="value"><?php echo Forum::totalTopics(); ?></div>
</div>
<div id="posts">
<div id="info">Posts</div>
<div id="value"><?php echo Forum::totalPosts(); ?></div>
</div>
<div id="comments">
<div id="info">Comments</div>
<div id="value"><?php echo Forum::totalComments(); ?></div>
</div>
<div id="latestuser">
<div id="info">Latest User</div>
<?php
$latestuser = Account::getLatestUser();
if ($latestuser == NULL)
$latestuser = 'none';
?>
<div id="value"><a href="profile.php?profile=<?php echo $latestuser; ?>"><?php echo $latestuser; ?></a></div>
</div>
</div>
</div>
</div>
</div>
</div>
<?php require 'footer.php' ?>
<!--
Forum Statistics
users
posts
comments
-->
<?php
require 'libraries/Account.php';
require 'libraries/cookies.php';
?>
<!DOCTYPE html>
<html>
<head>
<title>Event Horizon</title>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" type="text/css" href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css">
<script src="https://kit.fontawesome.com/d756906828.js" crossorigin="anonymous"></script>
<link href="https://fonts.googleapis.com/css?family=Source+Sans+Pro&display=swap" rel="stylesheet">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script>
<link rel="stylesheet" type="text/css" href="css/main.css">
<link rel="stylesheet" type="text/css" href="css/profile.css">
<link rel="stylesheet" type="text/css" href="css/forum.css">
<script>
var p = $(location).attr('pathname').split('/');
var pp = p[p.length-1].split('.');
var ppp = pp[0];
var pppp = ppp.substr(0,1).toUpperCase() + ppp.substr(1);
$(document).ready(function() {
if (pppp === 'Index') pppp = 'Home';
document.title = 'Event Horizon - ' + pppp + ' Page';
});
</script>
</head>
<body>
<div class="container-fluid col-md-12" style="background-color: #e8e8e8;">
<?php include 'nav.php'; ?>
<?php include('header.php');
include('libraries/Forum.php');
include('widget/Discord.php');
?>
<!-- FILLER -->
<?php
$discord = new Discord();
if (Account::IsLoggedIn()) {
?>
<!-- CONTENT -->
<div class="content">
<div class="welcomemessage"><p>Welcome Back, <?php echo Account::getData(Account::USERNAME); ?>!</p></div>
<!-- -->
<?php
} else {
?>
<!-- -->
<!-- BANNER -->
<div class="banner-container" style="background-image: url('images/logothing2.png');">
<div class="banner-container-inner">
<h1>EVENT HORIZON</h1>
<h2>A gaming and coding community</h2>
<!--<a class="btn" href="signup.php">JOIN THE COMMUNITY</a>-->
</div>
</div>
<!-- -->
<div class="row">
<div class="col-md-8 offset-md-2">
<div class="board">
<div class="row">
<div class="col-md-9" id="mainpanel">
<div id="content">
<!--
<div id="serverlist">
<div id="leftarrow"></div>
<div id="server">
<div id="slide">
<div id="discord">
<div id="image">
<img src="images/discordlogo.png" />
</div>
<div id="playercount">
<p><?php echo $discord->getUserCount(); ?> Members Online</p>
<p><?php echo $discord->getUsersInVoice(); ?> Members Talking</p>
</div>
<div id="button">
<?php
$link = $discord->getInviteLink();
if ($link == NULL)
$link = 'index.php';
?>
<a class="btn btn-primary" href="<?php echo $link; ?>">Join The Community</a>
</div>
</div>
</div>
<div id="slide">factorio</div>
<div id="slide">minecraft</div>
</div>
<div id="rightarrow"></div>
</div>
<script>
var currentIndex = 0;
var maxIndex = 2;
function hide(index) {
$('.board #slide').eq(index).css('display', 'none');
}
function show(index) {
$('.board #slide').eq(index).css('display', 'initial');
}
show(currentIndex);
$('#leftarrow').click(function() {
hide(currentIndex);
if (currentIndex <= 0)
currentIndex = maxIndex;
else
currentIndex--;
show(currentIndex);
});
$('#rightarrow').click(function() {
hide(currentIndex);
if (currentIndex >= maxIndex)
currentIndex = 0;
else
currentIndex++;
show(currentIndex);
});
</script>
-->
<?php
foreach (Forum::getHomepagePosts() as $p) {
$title = $p['title'];
$message = html_entity_decode($p['message']);
$message = str_replace('"', '\"', $message);
$message = str_replace('\'', '\"', $message);
$username = Account::getData(Account::USERNAME, $p['user_id']);
?>
<div id="post">
<div id="header">
<p><a href="Thread.php?post=<?php echo $p['id']; ?>"><?php echo $title; ?></a></p>
</div>
<div id="content">
<?php echo nl2br($message); ?>
<div class="btn btn-primary" id="creading">
<a href="Thread.php?post=<?php echo $p['id']; ?>">Continue Reading...</a>
</div>
</div>
<div id="footer">
<div id="accountName">
<p>by <a href="profile.php?profile=<?php echo $username; ?>"><?php echo $username; ?></a></p>
</div>
<div id="comments">
<p>Comments : <?php echo Forum::totalPostComments($p['id']); ?></p>
</div>
</div>
</div>
<?php
}
?>
</div>
</div>
<div class="col-md-3" id="sidepanel">
<div id="latestpost">
<div id="header">
<p>Recent Posts</p>
</div>
<div id="content">
<?php
foreach(Forum::getForumLatestPost() as $p) {
$username = Account::getData(Account::USERNAME, $p['user_id']);
?>
<div id="post">
<div id="avatarimage"><a href="profile.php?profile=<?php echo $username; ?>"><img src="<?php echo Account::getData(Account::AVATAR, $p['user_id']); ?>" /></a></div>
<div id="infoDiv">
<div id="title"><a href="Thread.php?post=<?php echo $p['id']; ?>"><?php echo $p['title']; ?></a></div>
<div id="name"><a href="profile.php?profile=<?php echo $username; ?>"><?php echo $username; ?></a></div>
</div>
</div>
<?php
}
?>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
<!-- -->
<?php
}
?>
</div>
<!-- -->
<?php include('footer.php'); ?>
<?php include 'header.php';
if (Account::isLoggedIn())
header('Location: index.php');
$remember = cookies::getCookie('remembermeusername');
function getValue($key) {
$url = $_SERVER['QUERY_STRING'];
$decoded = urldecode($url);
if (strpos($decoded, 'enc=true') !== false) {
$ar = explode('&', $decoded);
foreach ($ar as $a) {
$ex = explode('=', $a);
if ($ex[1] != NULL && $ex[0] === $key)
return $ex[1];
}
}
return '';
}
?>
<div class="content container text-center">
<div class="col-md-4 col-md-offset-4 loginform">
<form action="action.php" method="POST">
<label id="signinlabel">Sign In</label>
<div class="loginErrorMessage"><p><?php echo getValue('errormessage'); ?></p></div>
<div class="form-group">
<div class="icon-container">
<i class="fa fa-user icon"></i>
<input name="username" type="text" class="form-control icon-field" id="username" placeholder="Username" value="<?php if ($remember != NULL) echo $remember; ?>">
</div>
</div>
<div class="form-group">
<div class="icon-container">
<i class="fa fa-key icon"></i>
<input name="password" type="password" class="form-control" id="password" placeholder="Password">
</div>
</div>
<div class="form-group form-check rememeberme">
<input name="rememberme" type="checkbox" class="form-check-input remembermeinput">
<label class="form-check-label remembermelabel" for="exampleCheck1">Remember me</label>
</div>
<div class="fplink">
<a href="#">Forgot Password?</a>
</div>
<button name="loginaccount" type="submit" class="btn btn-primary">Submit</button>
</form>
</div>
</div>
<?php
if ($remember != NULL) {
?>
<script>$('.remembermeinput').prop('checked', 'true');</script>
<?php
}
if (getValue('errormessage') === '') {
?>
<script>
$('.loginErrorMessage').remove();
</script>
<?php
}
include 'footer.php'; ?>
<nav class="navbar navbar-expand-lg navbar-dark" style="background-color: #222831;">
<a class="navbar-brand" href="index.php">Event Horizon</a>
<button class="navbar-toggler" type="button" data-toggle="collapse" data-target="#navbarNavDropdown" aria-controls="navbarNavDropdown" aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse" id="navbarNavDropdown">
<ul class="navbar-nav">
<li class="nav-item">
<a class="nav-link" href="index.php">Home</a>
</li>
<li class="nav-item">
<a class="nav-link" href="forum.php">Forum</a>
</li>
<li class="nav-item dropdown">
<a class="nav-link dropdown-toggle" href="#" id="navbarDropdownMenuLink" role="button" data-toggle="dropdown" aria-haspopup="true" aria-expanded="false">
Repository
</a>
<div class="dropdown-menu" aria-labelledby="navbarDropdownMenuLink">
<a class="dropdown-item" target="_blank" href="https://github.com/scotth0828/EventHorizon">Site</a>
<a class="dropdown-item" target="_blank" href="https://github.com/Karutoh/ComplexRP">Gamemode</a>
</div>
</li>
<ul class="nav navbar-nav navbar-right">
<?php
if (!Account::isLoggedIn()) {
?>
<li class="nav-item"><a class="nav-link" href="login.php"><i class="fas fa-sign-in-alt"></i> Login</a></li>
<li class="nav-item"><a class="nav-link" href="signup.php"><i class="fas fa-user-plus"></i> Sign Up</a></li>
<?php
} else {
?>
<li class="nav-item dropdown dropdown-user">
<a class="nav-link dropdown-toggle" href="#" id="navbarDropdownMenuLink" role="button" data-toggle="dropdown" aria-haspopup="true" aria-expanded="false">
<div class="dropdown-user-menu-item">
<?php
$id = Account::getData(Account::ID);
$username = Account::getData(Account::USERNAME);
$avatar = Account::getData(Account::AVATAR);
echo '<img id="avatar" src="'.$avatar.'"></img>';
echo '<p>'.$username.'</p>';
?>
</div>
</a>
<div class="dropdown-menu" aria-labelledby="navbarDropdownMenuLink">
<a class="dropdown-item" target="" href="profile.php">Profile</a>
<a class="dropdown-item" target="" href="action.php?code=signout">Sign Out</a>
</div>
</li>
<?php
}
?>
</ul>
</ul>
</div>
</nav>
<script>
$(document).ready(function() {
$('.nav-link').each(function() {
var p = $(location).attr('pathname').split('/');
var loc = p[p.length-1];
if (loc === $(this).attr('href')) {
$(this).css('color', 'white');
}
});
});
</script>
<?php
require 'header.php';
$userprofile = false;
$avatar = 'images/default_avatar.jpg'; // default avatar
$tag = '@default';
$bio = 'deafault bio description';
$userfollowed = '';
DB::createFollowerTable();
DB::createPostTable();
if (isset($_GET['profile']) == NULL) {
if (Account::isLoggedIn()) {
$userprofile = true;
$userfollowed = $username;
$avatar = Account::getData(Account::AVATAR);
$tag = '@' . Account::getData(Account::USERNAME);
} else {
header('Location: index.php');
die();
}
} else {
$username = $_GET['profile'];
$id = Account::getIDFromType(Account::USERNAME, $username);
if (Account::getData(Account::ID) == $id) {
$userprofile = true;
$userfollowed = $username;
}
else {
$userfollowed = $username;
}
if ($id != NULL) {
$avatar = Account::getData(Account::AVATAR, $id);
$tag = '@' . Account::getData(Account::USERNAME, $id);
} else {
header('Location: index.php');
die();
}
}
if (isset($_POST['createpost'])) {
$message = $_POST['textarea'];
$m = nl2br($message);
$p = preg_replace('~[\r\n]+~', '', $m);;
$post = htmlentities($p);
$date = date('Y-m-d H-i-s');
$id = Account::getData(Account::ID);
echo DB::setData('posts', array('PostDate'=>$date, 'post'=>$post, 'user_id'=>$id, 'homepage'=>'0'));
}
?>
<!-- CONTENT -->
<div class="pcontent">
<div class="profilebanner">
<div class="profileavatar noselect">
<img class="noselect" src="<?php echo $avatar; ?>">
</div>
</div>
<div class="profilecontent">
<div class="container">
<div class="row">
<div class="col-md-4">
<div class="tag"><?php echo $tag; ?></div>
<?php if ($userprofile) { ?>
<form action="editprofile.php" method="POST">
<button class="btn btn-primary editprofile">Edit Account</button>
</form>
<?php } ?>
<div class="followfollowingcount">
<p><?php echo count(Account::getFollowers(Account::getIDFromType(Account::USERNAME, $userfollowed))); ?> Followers</p>
<p> | </p>
<p><?php echo count(Account::getFollowing(Account::getIDFromType(Account::USERNAME, $userfollowed))); ?> Following</p>
</div>
<?php if (!$userprofile && Account::isLoggedIn()) { ?>
<div class="followblock">
<?php
$f = Account::isFollowing(Account::getIDFromType(Account::USERNAME, $userfollowed));
if ($f != Account::BLOCKED) { ?>
<form action="action.php" method="POST">
<input type="hidden" name="user" value="<?php echo $userfollowed; ?>">
<button name="follow" class="btn btn-primary followbtn">
<?php
if ($f != Account::FOLLOWING)
echo 'Follow';
else
echo 'UnFollow';
?>
</button>
</form>
<?php } ?>
<form action="action.php" method="POST">
<input type="hidden" name="user" value="<?php echo $userfollowed; ?>">
<button name="block" class="btn btn-primary blockbtn">
<?php
if ($f != Account::BLOCKED)
echo 'Block';
else
echo 'UnBlock';
?>
</button>
</form>
</div>
<?php } ?>
<div class="bio"><?php echo $bio; ?></div>
</div>
<div class="col-md-8">
<div class="profile-posts-container">
<?php
if (Account::isLoggedIn()) {
?>
<form action="" method="POST" class="form-group">
<textarea name="textarea" class="form-control" rows="3"></textarea>
<!--<button name="createpost" class="btn btn-primary">Post Message</button>-->
<input type="submit" name="createpost" class="btn btn-primary" id="button" value="Post Message">
</form>
<?php
}
?>
<div class="profile-posts"></div>
</div>
</div>
</div>
</div>
</div>
</div>
<!-- -->
<?php
$posts = Account::getLatestPosts(Account::getIDFromType(Account::USERNAME, $userfollowed), 5);
$latestPostID = $posts[count($posts)-1]['id'];
for ($i = 0; $i <= count($posts)-1; $i++) {
$message = html_entity_decode($posts[$i]['post']);
$message = str_replace('"', '\"', $message);
$message = str_replace('\'', '\"', $message);
$date = date("F j, Y", strtotime($posts[$i]['PostDate']));
Account::createPost('.profile-posts', $i, $date, $message, $posts[$i]['user_id']);
}
?>
<script>
var info = {};
info['currentPostID'] = <?=json_encode($latestPostID);?>;
function check() {
$.ajax({
type: 'POST',
url: 'c.php',
dataType: 'json',
data: info,
success: function(data){
info['currentPostID'] = parseFloat(data.latestID);
console.log(info['currentPostID']);
}
});
}
setInterval(check, 1000);
</script>
<?php include('footer.php'); ?>
<?php include 'header.php';
DB::createUserTable();
if (Account::isLoggedIn())
header('Location: index.php');
function getValue($key) {
$url = $_SERVER['QUERY_STRING'];
$decoded = urldecode($url);
if (strpos($decoded, 'enc=true') !== false) {
$ar = explode('&', $decoded);
foreach ($ar as $a) {
$ex = explode('=', $a);
if ($ex[1] != NULL && $ex[0] === $key)
return $ex[1];
}
}
return '';
}
?>
<div class="content container text-center">
<div class="col-md-4 col-md-offset-4 loginform">
<form action="action.php" method="POST">
<label id="signinlabel">Sign Up</label>
<div class="loginErrorMessage"><p><?php echo getValue('errormessage'); ?></p></div>
<div class="form-group">
<div class="icon-container">
<i class="fa fa-user icon"></i>
<input name="username" type="text" class="form-control" id="username" placeholder="Username" value="<?php echo getValue('username'); ?>">
</div>
</div>
<div class="form-group">
<div class="icon-container">
<i class="fas fa-envelope-square icon"></i>
<input name="email" type="email" class="form-control" id="email" placeholder="Email" value="<?php echo getValue('email'); ?>">
</div>
</div>
<div class="form-group">
<div class="icon-container">
<i class="fa fa-key icon"></i>
<input name="password" type="password" class="form-control" id="password" placeholder="Password">
</div>
</div>
<div class="form-group">
<div class="icon-container">
<i class="fa fa-key icon"></i>
<input name="confirmpassword" type="password" class="form-control" id="confirmpassword" placeholder="Confirm Password">
</div>
</div>
<div class="form-group">
<div class="icon-container">
<i class="far fa-calendar-alt icon"></i>
<input name="birthdate" type="date" class="form-control" id="birthdate" placeholder="birthdate" value="<?php echo getValue('birthdate'); ?>">
</div>
</div>
<button name="register" type="submit" class="btn btn-primary">Register</button>
</form>
</div>
</div>
<?php
if (getValue('errormessage') === '') {
?>
<script>
$('.loginErrorMessage').remove();
</script>
<?php
}
include 'footer.php'; ?>